Web サイトでライトモードとダークモードを切り替えることができるようになっています。私個人も、ダークモードを利用しています。今回はこれを NextUI で実装する手順を確認していきます。
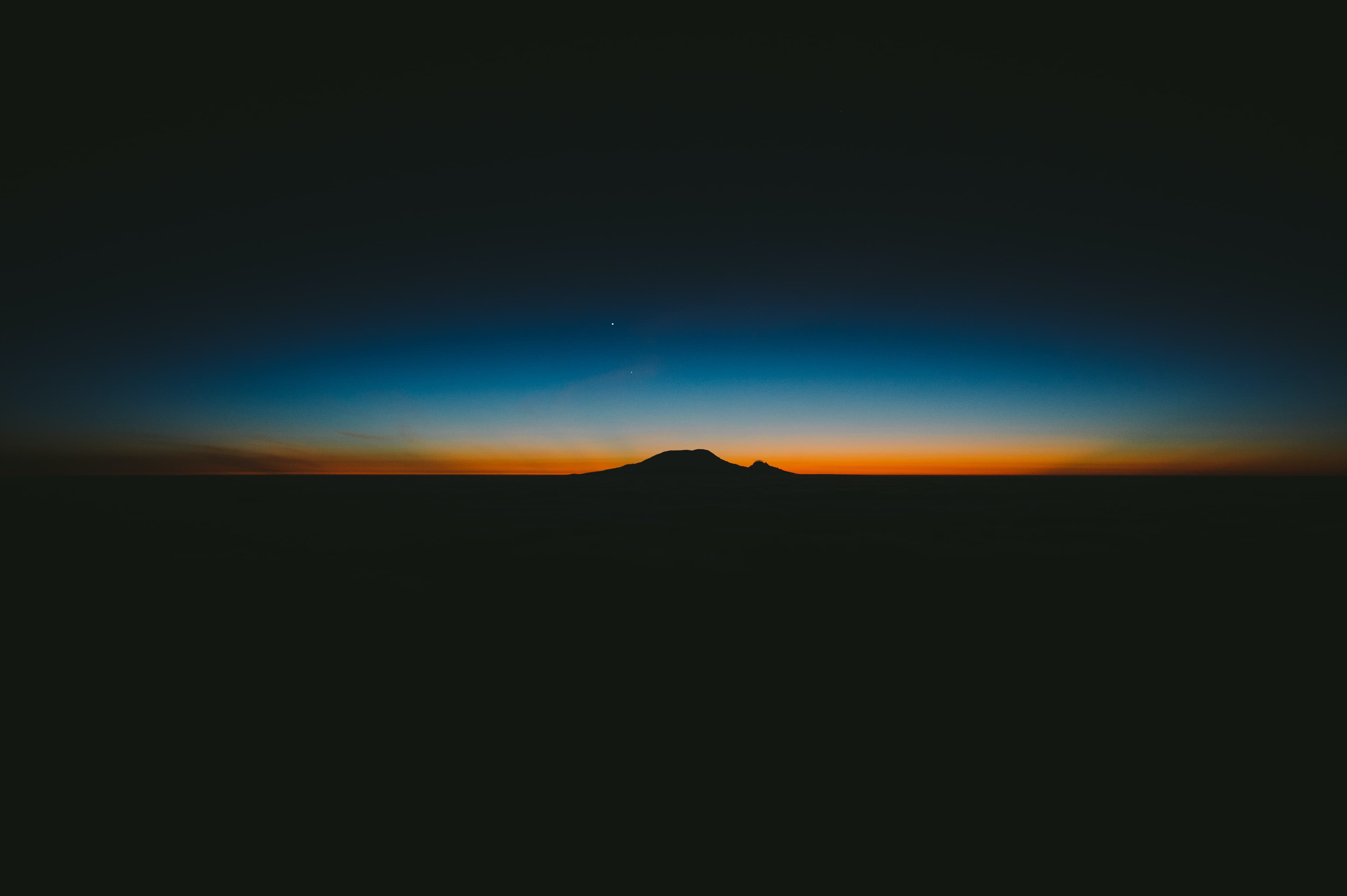
ダークモードを実装する
まず最初に、プロジェクトでダークモードを利用するためのモジュールを以下のようにインストールします。
PowerShell
npm install next-themes
インストールをした後、app\providers.tsx のページに対して、next-themes を利用して書き換えます。
TypeScript
// app/providers.tsx
"use client";
import { NextUIProvider } from "@nextui-org/react";
import { ThemeProvider as NextThemesProvider } from "next-themes";
export function Providers({ children }: { children: React.ReactNode }) {
return (
<NextUIProvider>
<NextThemesProvider attribute="class" defaultTheme="light">
{children}
</NextThemesProvider>
</NextUIProvider>
);
}
続いて、app\globals.css のファイルに記載されている Class を一通り削除して、以下の行のみとします。
CSS
@tailwind base;
@tailwind components;
@tailwind utilities;
最後に、表示するボタンを増やすために app\page.tsx に以下のようにボタンを並べます。
TypeScript
<div className="m-8 flex flex-wrap gap-4 items-center">
<Button color="default">Default</Button>
<Button color="primary">Primary</Button>
<Button color="secondary">Secondary</Button>
<Button color="success">Success</Button>
<Button color="warning">Warning</Button>
<Button color="danger">Danger</Button>
</div>
この状態でアクセスをすると、以下のようにライトモードでアクセスができます。
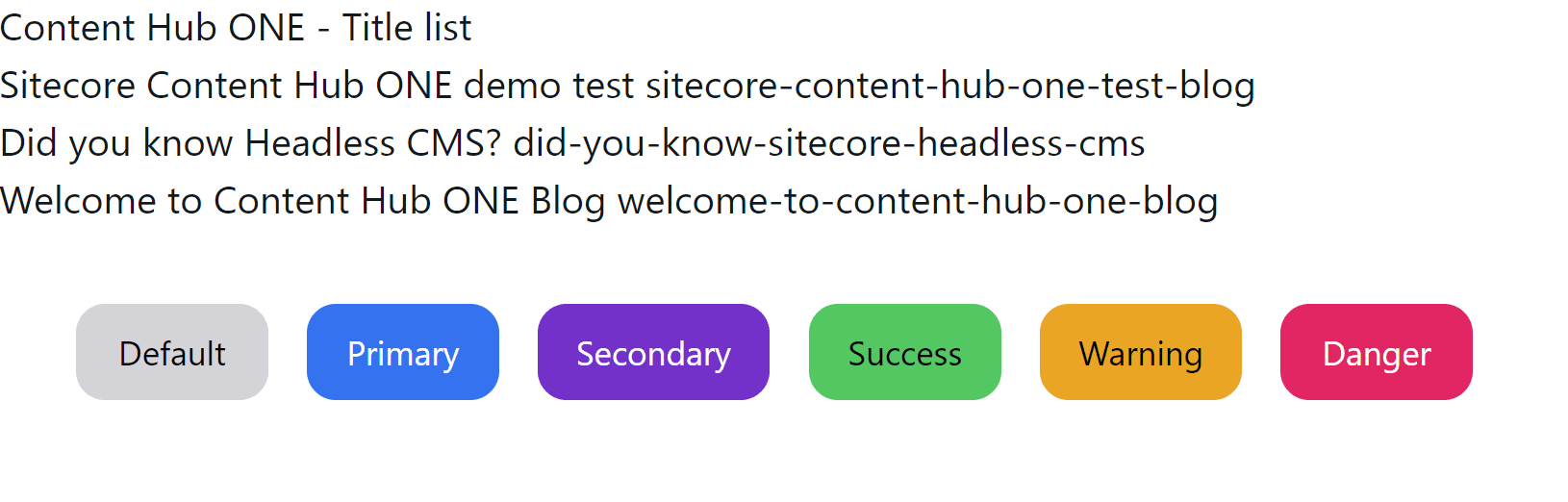
切り替えボタンの追加
ボタンのサンプルコードも上記のページにあります。今回は、components\button\ThemeSwitcher.tsx というファイルを作成して、以下のようなコードを用意しました。
TypeScript
// components/button/ThemeSwitcher.tsx
"use client";
import { useTheme } from "next-themes";
import { useEffect, useState } from "react";
import { BsSun, BsMoon } from "react-icons/bs";
export function ThemeSwitcher() {
const [mounted, setMounted] = useState(false);
const { theme, setTheme } = useTheme();
useEffect(() => {
setMounted(true);
}, []);
const handleSetTheme = () => {
setTheme(theme === "light" ? "dark" : "light");
};
if (!mounted) return null;
return (
<div>
<button className="block p-1 rounded-full" onClick={handleSetTheme}>
{theme === "light" ? (
<BsMoon className="w-5 h-5" />
) : (
<BsSun className="w-5 h-5" />
)}
</button>
</div>
);
}
このコードでは react-icons を利用しているため、以下のようにプロジェクトに追加してください。
PowerShell
npm install react-icons
ボタンをクリックすると切り替わるようになりました。
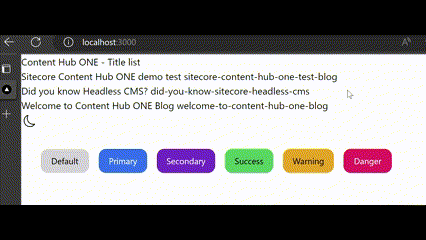
まとめ
NextUI は最近リリースされただけに、ダークモードを簡単に利用できるようになっています。企業のサイトとかでは不要だと思うこともありますが、訪問する人が読みやすいドキュメントサイトにしたい、というのには便利な機能です。